- Python Code For Dynamic Key Generation 1
- C Code Generation Using Python
- Python Dynamic Programming
- Python Dynamic Import
- Python Code For Dynamic Key Generation Download
Cog is a simple code generation tool written in Python. We use it or itsresults every day in the production of Kubi.
Kubi is a collaboration system embodied in a handful of different products.We have a schema that describes the representation of customers'collaboration data: discussion topics, documents, calendar events, and so on.This data has to be handled in many ways: stored in a number of differentdata stores, shipped over the wire in an XML representation, manipulated inmemory using traditional C++ objects, presented for debugging, and reasonedabout to assess data validity, to name a few.
Apr 22, 2019 The generation of all the keys used is made in the method generatekeys and substitute apply the SBOX permutation. The main method is run which is called by both encrypt and decrypt but in a different mode. This method do basically all the stuff, it loop throught all the blocks and for each do the 16th rounds. Generating Keys for Encryption and Decryption.; 3 minutes to read +7; In this article. Creating and managing keys is an important part of the cryptographic process. Symmetric algorithms require the creation of a key and an initialization vector (IV). The key must be kept secret from anyone who should not decrypt your data. The given master key is stretched and expanded by PKBDF2-HMAC(SHA256) using the salt from 1), to generate the AES key, HMAC key and IV (initialization vector for CBC). The given message is encrypted with AES-128 using the AES key and IV from step 2), in CBC mode and PKCS#7 padding. Note: the above code is perfectly acceptable for expository purposes, but remember that in Python 2 firstn is equivalent to the built-in xrange function, and in Python 3 range is an immutable sequence type. The built-ins will always be much faster. Generate a random permutation of elements from range L, R (Divide and Conquer) Minimum number of given operations required to convert a permutation into an identity permutation Generate a graph using Dictionary in Python.
We needed a way to describe this schema once and then reliably produceexecutable code from it.
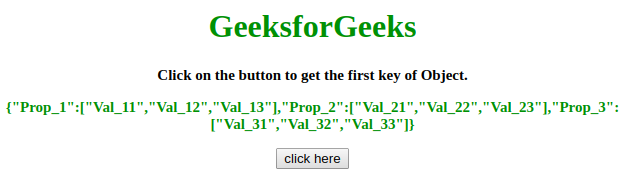
Our first implementation of this schema involved a fractured collection ofrepresentations. The XML protocol module had tables describing theserialization and deserialization of XML streams. The storage modules hadother tables describing the mapping from disk to memory structures. Thevalidation module had its own tables containing rules about which propertieshad to be present on which items. The in-memory objects had getters andsetters for each property.
It worked, after a fashion, but was becoming unmanageable. Adding a newproperty to the schema required editing ten tables in different formats inas many source files, as well as adding getters and setters for the newproperty. There was no single authority in the code for the schema as awhole. Different aspects of the schema were represented in differentways in different files.

We tried to simplify the mess using C++ macros. This worked to a degree, butwas still difficult to manage. The schema representation was hampered by thesimplistic nature of C++ macros, and the possibilities for expansion wereextremely limited.
The schema tables that could not be created with these primitive macros werestill composed and edited by hand. Changing a property in the schema stillmeant touching a dozen files. This was tedious and error prone. Missing oneplace might introduce a bug that would go unnoticed for days.
It was becoming clear that we needed a better way to manage the propertyschema. Not only were the existing modifications difficult, but new areas ofdevelopment were going to require new uses of the schema, and new kinds ofmodification that would be even more onerous.
We'd been using C++ macros to try to turn a declarative description of theschema into executable code. The better way to do it is with codegeneration: a program that writes programs. We could use a tool to read theschema and generate the C++ code, then compile that generated code into theproduct.
We needed a way to read the schema description file and output pieces of codethat could be integrated into our C++ sources to be compiled with the rest ofthe product.
Rather than write a program specific to our problem, I chose instead to writea general-purpose, although simple, code generator tool. It would solve theproblem of managing small chunks of generator code sprinkled throughout alarge collection of files. We could then use this general purpose tool tosolve our specific generation problem.
The tool I wrote is called Cog. Its requirements were:
- We needed to be able to perform interesting computation on the schema tocreate the code we needed. Cog would have to provide a powerful languageto write the code generators in. An existing language would make it easierfor developers to begin using Cog.
- I wanted developers to be able to change the schema, and then run the toolwithout having to understand the complexities of the code generation. Cogwould have to make it simple to combine the generated chunks of code withthe rest of the C++ source, and it should be simple to run Cog to generatethe final code.
- The tool shouldn't care about the language of the host file. We originallywanted to generate C++ files, but we were branching out into otherlanguages. The generation process should be a pure text process, withoutregard to the eventual interpretation of that text.
- Because the schema would change infrequently, the generation of code shouldbe an edit-time activity, rather than a build-time activity. This avoidedhaving to run the code generator as part of the build, and meant that thegenerated code would be available to our IDE and debugger.
The language I chose for the code generators was, of course, Python. Itssimplicity and power are perfect for the job of reading data files andproducing code. To simplify the integration with the C++ code, the Pythongenerators are inserted directly into the C++ file as comments.
Cog reads a text file (C++ in our case), looking for specially-markedsections of text, that it will use as generators. It executes those sectionsas Python code, capturing the output. The output is then spliced into thefile following the generator code.
Because the generator code and its output are both kept in the file, there isno distinction between the input file and output file. Cog reads and writesthe same file, and can be run over and over again without losing information.
Cog processes text files, converting specially marked sections of the fileinto new content without disturbing the rest of the file or the sectionsthat it executes to produce the generated content.Zoom in
In addition to executing Python generators, Cog itself is written in Python.Python's dynamic nature made it simple to execute the Python code Cog found,and its flexibility made it possible to execute it in a properly-constructedenvironment to get the desired semantics. Much of Cog's code is concernedwith getting indentation correct: I wanted the author to be able to organizehis generator code to look good in the host file, and produce generated codethat looked good as well, without worrying about fiddly whitespace issues.
Python Code For Dynamic Key Generation 1
Python's OS-level integration let me execute shell commands where needed. Weuse Perforce for source control, which keeps files read-only until they needto be edited. When running Cog, it may need to change files that thedeveloper has not edited yet. It can execute a shell command to check outfiles that are read-only.
Lastly, we used XML for our new property schema description, and Python'swide variety of XML processing libraries made parsing the XML a snap.
C Code Generation Using Python
Here's a concrete but slightly contrived example. The properties aredescribed in an XML file:
Python Dynamic Programming
We can write a C++ file with inlined Python code:
After running this file through Cog, it looks like this:
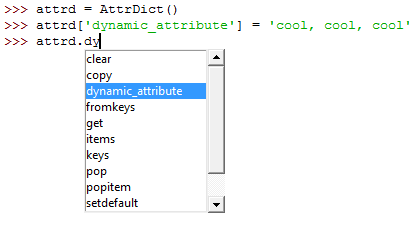
Python Dynamic Import
The lines with triple-brackets are marker lines that delimit the sections Cogcares about. The text between the [[[cog and ]]] lines is generator Pythoncode. The text between ]]] and [[[end]]] is the output from the last run ofCog (if any). For each chunk of generator code it finds, Cog will:
- discard the output from the last run,
- execute the generator code,
- capture the output, from the cog.outl calls, and
- insert the output back into the output section.
In a word, great. We now have a powerful tool that lets us maintain a singleXML file that describes our data schema. Developers changing the schema havea simple tool to run that generates code from the schema, producing outputcode in four different languages across 50 files.
Where we once used a repetitive and aggravating process that was inadequateto our needs, we now have an automated process that lets developers expressthemselves and have Cog do the hard work.
Python's flexibility and power were put to work in two ways: to develop Cogitself, and sprinkled throughout our C++ source code to give our developers apowerful tool to turn static data into running code.
Although our product is built in C++, we've used Python to increase ourproductivity and expressive power, ease maintenance work, and automateerror-prone tasks. Our shipping software is built every day with Pythonhard at work behind the scenes.
More information, and Cog itself, is available athttp://www.nedbatchelder.com/code/cog
Python Code For Dynamic Key Generation Download
Ned Batchelder is a professional software developer who struggles along withC++, using Python to ease the friction every chance he gets. A previousproject of his,Nat's World *was the subject of an earlier Python Success Story.*